22. Functions, IIa: Definition and arguments¶
Conceptually, a function is a piece of a program, a set of instructions arranged in order to carry out one or more well-defined tasks. We distinguish two categories of functions in Python, the predefined functions and the user-written ones:
The predefined functions are functions directly integrated into the standard library (what are called built-in functions) and into modules of the Python system (which we saw in discussion importing modules).
User functions are written either by the current user or by other users. These can be placed directly in a program/script, or imported, as well.
22.1. Some predefined functions in python¶
The function print()
: As we have already seen, this function has the role of
displaying on the screen the values of the objects specified as
arguments:
print("Good morning", "to", "all of you")
x=12
print(x)
y=[1, "Monday","12", 5, 3, "test value"]
print(y)
You can replace the default separator (space) by any other character (or
even with no characters), thanks to the argumentsep
:
print("Good morning", "to", "all of you", sep= "****")
print(""Good morning", "to", "all of you", sep= "")
The
input ()
function: The ** input () ** function allows you to give the user control so that he enters the value of a given argument:
first_name = input("Enter you first name : ")
print("Bonjour,", first_name)
print("Please enter any positive number: ", end=" " )
ch = input()
num = int(ch) # converting string to integer
print("The square of", num, "is", num**2)
Note
Note that the input()
function always returns a
string. If you want the user to enter a numeric value, so
you will need to convert the value input (which will
therefore be of type string anyway) into a numerical value
of the type you suitable, via the built-in functions
int()
(if you expect an integer) or float()
(if you
are expecting a real one).
22.2. User-defined functions¶
22.2.1. Defining a function¶
When there is not an available function to perform a task, you can write your own functions. The simplest functions have the following format in Python:
def <function name>():
<function body>
# define a function
def do_nothing():
s = "I don't do much"
# call a function
do_nothing()
However, this often isn’t very useful since we haven’t returned any
values from this file. Note that if you don’t return anything from a
function in Python, you implcitly have returned the special None
singleton.
def do_nothing():
s = "I don't do much"
output = do_nothing()
type(output)
... which produces:
NoneType
To return vaulues that you computed locally in the function body, use
the return keyword. To define a function in Python, we use the
keyword def
to specify the name of the function. The basic syntax
for defining a function is as follows:
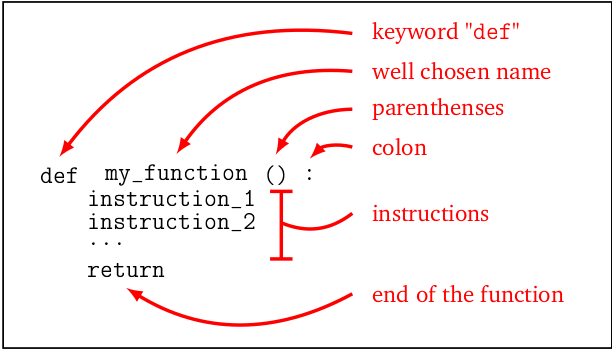
22.2.2. Definition of a function without arguments¶
def hello_function():
print('Hello World, it is me. I am a function.')
You call it doing:
hello_function()
Hello World, it is me. I am a function.
The example below illustrates the definition of a simple function without arguments and a return instruction.
def return_me():
s = 'Hello World, it is me. I am a function.'
return s
return_me()
'Hello World, it is me. I am a function.'
The output looks the same for the functions but remember, there is a difference between what Python displays and what Python knows about what it displays. So if we set:
r = hello_function()
t = return_me()
# We can see that
type(r) # returns the argument of the argument of the print function
type(t) # a string
Hello World, it is me. I am a function.
str
22.2.3. Definition of a function with arguments¶
Computer scientists' functions acquire their full potential with:
an input, which groups together variables that serve as arguments,
an output, which is a result returned by the function (and which often will depend on the arguments entry).
22.2.3.1. Function with one argument¶
Functions, may be defined to take parameters or arguments.
def <function name>(<argument>):
<function body>
return <local variable 1>
The function name, arguments, and return are jointly known as the
function signature since the uniquely define the function’s
interface. Using a function is done by placing parentheses ()
after the function name after you have defined it. This is known as
calling the function. If the function requires arguments, the values
for these arguments are inside of the parentheses
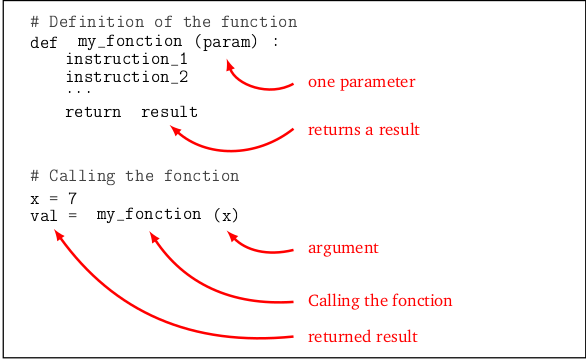
def square(x):
sqr = x * x
return sqr
square(2)
4
22.2.3.2. Function with (multiple) arguments/(multiple) return values,¶
There may be multiple input arguments, there may be multiple output results. That is, functions may be defined such that they have multiple arguments or multiple return values:
def <function name>(<arg1>, <arg2>, ...):
<functiom body>
return <var1> , <var2>, ...
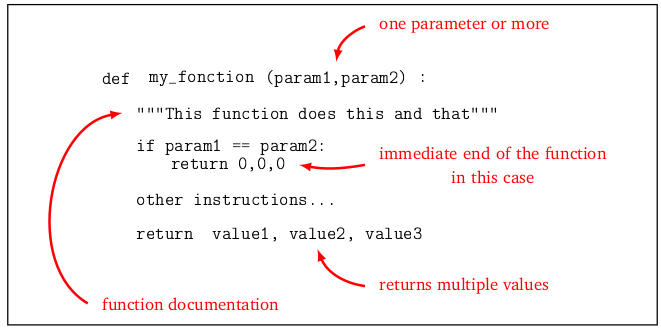
Comments and docstring
Anything after the pound sign # is a comment and is ignored by Python:
# Main loop while r != 0: # As long as r is non zero r = r - 1 # Reduce by 1
You can describe what a function does by starting with a docstring, that is to say adescription, surrounded by three quotation marks:
def produit(x,y): """ Calculate the product of two numbers Input: two numbers x and y Output: the product of x by y""" p = x * y return p
Here is an example of a function with two arguments and two outputs.
def sum_prod(x,y):
""" Computes the sum and product of two numbers. """
#Sum
S = x + y
# Product
P = x*y
return S, P # Returns the results
# Calling the function
Sum, prod = sum_prod(3,7) # Results
# Display
print("Sum :",Sum)
print("Product :",prod)
Sum : 10
Product : 21
22.2.3.3. Function with a variable number of arguments¶
It might happen that one does not know in advance the exact number of input arguments a function will take. That is, functions may be defined such that they have a variable number of arguments:
def <function name>(*args):
<functiom body>
return <var1> , <var2>, ...
Here is an example of such function which takes a variable number of arguments and return their sum and their product.
def sum_prod(*args):
""" Computes the sum and product of its arguments. """
#Sum
S = 0
# Product
P = 1
for value in args:
S += value
P *= value
return S, P # Returns the results
# Calling the function
Sum, Prod = sum_prod(3,7,1,2) # Results
# Display
print("Sum :",Sum)
print("Product :",Prod)
Sum : 13
Product : 42
22.2.3.4. Keyword Arguments¶
In Python, functions also support options default values for arguments. Arguments with an associated default are called keyword arguments. If this function is then called without one of these arguments being pesent the default value is used. All keyword arguments must come after normal arguments in the function definition:
def <function name>(<arg1>, <arg2>, <arg3>=<arg3 default>, <arg4>=<arg4 default>, ...):
<function body>
return <rtn>
def add_space(s, t="Mom"):
return s + " " + t
print(add_space("Hello"))
print(add_space("Morning", "Dad"))
Hello Mom
Morning Dad
You can also call any functions with their arguments, regular and keyword, with their argument names explicitly in the call. This uses equal signs in the same way that keyword arguments are defined.
print(add_space(s="Hello"))
print(add_space(s="Morning", t="Dad"))
Hello Mom
Morning Dad
If you have many keyword arguments, then they may be out of order in function call as long as they are explicit.
def f(x=1, y=2, z=3):
return 2*x**3 + 42*y - z
f(y=17, z=15, x=2)
715
Warning: be careful with mutable containers and default values. The value changes with every function call.
def add_to_list(val, seq=[]):
seq.append(val)
return seq
add_to_list(42)
[42]
add_to_list(16)
[42, 16]
add_to_list(39)
[42, 16, 39]
22.3. Practice¶
Write a Python function to find the maximum of three numbers.
Write a Python function to sum all the even numbers in a list.
Write a Python function to multiply all the odd numbers in a list.
Write a Python function to calculate the factorial of a a non-negative integer.
Write a Python function to check whether a number is in a given range.
Write a Python function that takes
as argument and returns
computed as
#. Write a Python function f(n)
for computing the element in the
sequence
. Call the function for
and write
out the result.
Write a Python function for evaluating the mathematical function
and its derivative
. Note: the function returns 2 values.
Write a Python function for evaluating the mathematical function
Write a Python function for evaluating the mathematical function
Write a Python function to convert a temperature given in degrees Fahrenheit to its equivalent in degrees Celsius. You can assume that
, where
is the temperature in °C and
is the temperature in °F.
Write a Python function that takes a number as a parameter and checks if the number is prime or not.
Write a function that displays the n first terms of the Fibonacci sequence, defined by: