23. Functions, IIb: Scope of variables¶
23.1. Local variable¶
Here is a very simple function which takes as input a number and returns the number increased by one.
def my_function(x):
x = x + 1
return x
Of course my_function (3) returns 4.
If the value of y is 5, then my_function (y) returns 6. But beware, the value of y has not changed, it is always 5.
Here is the problematic situation that must be understood:
x = 7
print(my_function(x))
print(x)
The variable
x
is initialized to 7.Calling the my_function (x) function is therefore the same as my_function (7) and returns logically 8.
What is the value of the variable x at the end? The variable x is unchanged and always equals 7! Even though there was in between an instruction
x = x + 1
. This statement changed the x inside the function, but not the x outside the function.
x = 7
print(my_function(x))
print(x)
8
7
Variables defined inside a function are called local variables. They do not exist in outside the function.
If a variable in a function has the same name as a variable in the program (such as the x in the example above), there are two separate variables; the local variable only exists in the function.
23.2. Global variable¶
A global variable is a variable that is defined for the entire program. It is usually not recommended to use such variables, but it can be useful in some cases. Let’s see an example. We declare the global variable, here the gravitational constant, at the start of the program as a variable classic:
gravitation = 9.81
The content of the gravitation variable is now accessible everywhere. On the other hand, if we wish change the value of this variable in a function, we must specify to Python that we are aware to modify a global variable! For example for calculations on the Moon, it is necessary to change the gravitational constant which is much more lower there.
def on_the_moon():
global gravitation # Yes, I want to modify this global variable!
gravitation = 1.625 # New value for the whole program
...
To understand the scope of the variables, you can color the global variables of a function in red, and local variables with one color per function. The following small program defines a function that adds one, and another that calculates the double:
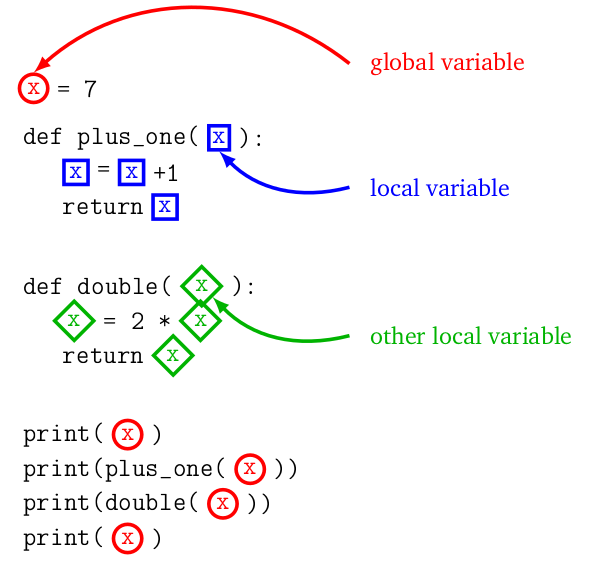
The program first displays the value of x, so 7, then it adds one to 7, so it displays 8, then it displays the double of x, so 14. The global variable x has never changed, so the last display of x is still 7. Kindly check that.
Describe the scope of the variables a, b, c and d in this example:
23.3. Practice¶
Consider the code below.
def doubled(a):
b = a * 2
return b
c = 5
if c > 2:
d = doubled(5)
print(d)
What is the lifetime of these variables? When will they be created and destroyed?
Can you guess what would happen if we were to assign c a value of 1 instead?
Why would this be a problem? Can you think of a way to avoid it?
What does the following code print?
n = 30
def my_function1():
n = 100
return n
print(my_function1(),n)
What does the following code print?
n = 30
def my_function2():
global n
n = 100
return n
print(my_function2(),n)
What does the following code print?
from math import pi
def some_function():
global pi
pi = 1
some_function()
print( pi )